Classes#
Overview#
Classes are essential part of object-oriented programming, by allowing the creation of functional “code components” for your program. The class
defines the attributes (data) and behaviors (methods/functions) of the instances of a class.
Once you define a class, you can create multiple objects, known as instances of a class, and these objects come with certain characteristics. For instance, str
class is a built-in class and when you create an instance of this class (e.g., my_string = “amazingABE”), this variable “my_string” is a string object and contains inherent methods for string manipulation, such as concatenation, slicing, replacing characters, and more.
General syntax
class ClassName:
# Constructor
def __init__(self, parameter1):
self.parameter1 = parameter1
# Methods (functions inside the class)
def method1(self):
# Code for method1
Does it sound complicated? Let’s start with simple class.
#Create a class named MyMajor, with a property named x:
class MyMajor:
major = 'Biomedical Engineering'
year = 'junior'
# Create Object
p1 = MyMajor() # Create an object named p1, and print the major by accessing the class attribute
print(p1.major)
Biomedical Engineering
This class is simple (and not mega useful) but it shows you the basic structure. Let’s clarify the difference of class and object:
Classes vs Objects#
Class:
A class is a template for creating objects
A class defines the attributes (data) and behaviors (methods) for the objects of this class
A class contains related data and methods into a single unit
Object (or instance):
An object is an instance of a class
Objects can have different values, but share the same methods
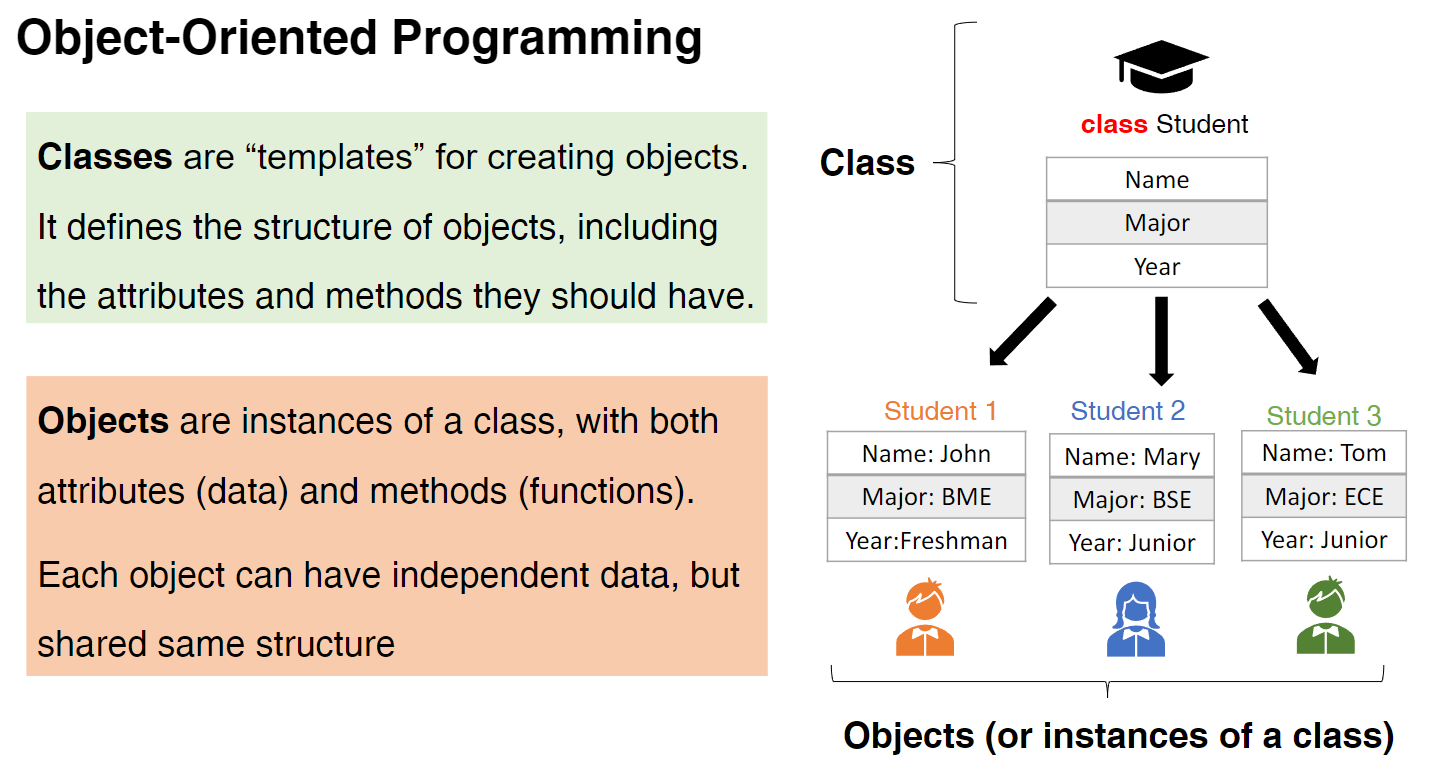
Create Multiple Objects#
The interesting aspect of Python Class is to reuse and create multiple objects from a single class.
init#
Let’s add another element of class: __init__()
function. The __init__()
function defines the object attributes and is always executed when the class is being initiated. It is automatically called when you create a new object (instance) of a class.
Also, self
parameter is just a convention that helps you to access and modify the object attributes.
class Student:
def __init__(self, name, major, year):
self.name = name
self.major = major
self.year = year
msu_student = Student("you", "ABE", 2023)
print(msu_student.name)
print(msu_student.major)
you
ABE
Object Methods#
A class can have inherent functions, known as object methods, and these functions can access and modify the attributes of the objects.
In other words, object methods are regular functions inside the class, and the self
parameter is used to access the attributes and methods of the instance.
# create a class
class Student:
def __init__(self, name, major, enroll_year):
self.name = name
self.major = major
self.enroll_year = enroll_year
# method to calculate area
def calculate_graduation(self):
print("Expected graduation year =", self.enroll_year + 4) # The self parameter is used to access variables that belongs to the class.
msu_student = Student("you", "ABE", 2023)
msu_student.calculate_graduation()
Expected graduation year = 2027
Important
self
parameter is named self by convention, but you can call it whatever you prefer as long as it is the first parameter of any function in the class