Variables#
Overview#
In your first code, you wrote a simple print()
, but python is way more excited than this. You can use variables to store values, and they act as boxes that stores information to be referenced throughout your program.
When you want to create a variable, you choose a name for the variable and assigning a value with assignment operator (=). Variables can store various types of data (numbers, strings, lists, etc) and we will discuss them later.
Variable names#
It’s good practice to use descriptive (but not long) names for variables to enhance code readability. Once you create a variable, you can access the value of a variable by simply referring to its name in your code. Let’s do it! Open the code editor, create a new example.py file, and write the below code:
# Creating variables and assigning values
lake_name = "Lake Erin"
# Accessing and using variable values
print("What is the lake name?", lake_name)
depth_ft = 279
print("What is the lake depth:", depth_ft)
min_width_mi = 91.0
print("What is the minimum lake width:", min_width_mi)
What is the lake name? Lake Erin
What is the lake depth: 279
What is the minimum lake width: 91.0
Instead of running this code in the Anaconda command prompt, you can use the Python Interpreter in PyCharm (or Spyder/VScode) to run this code.
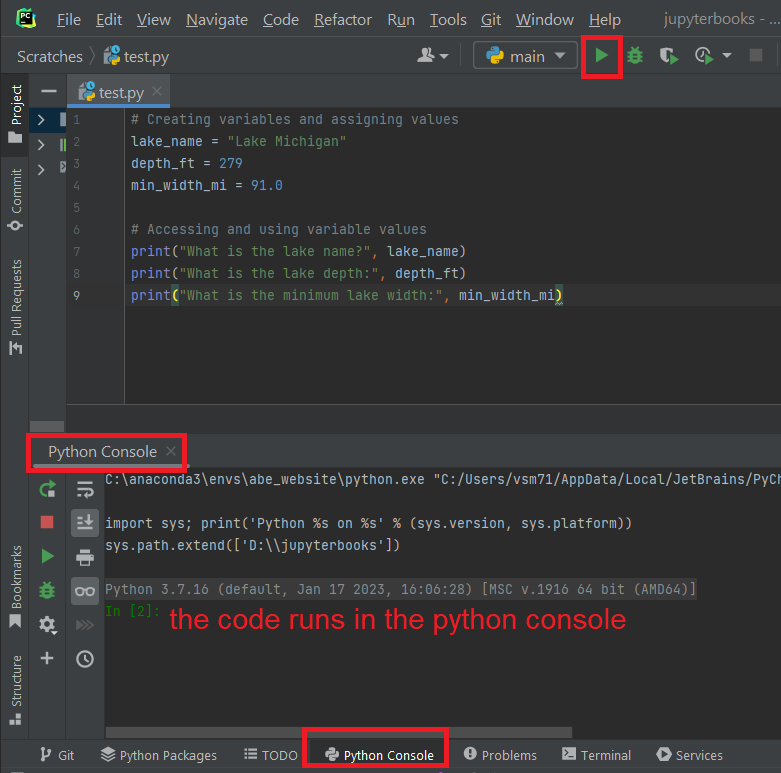
# Modifying variable values
lake_name = 'Lake Pontchartrain'
depth_ft = 65
# Updated values
print("Updated Name:", lake_name)
print("Updated depth:", depth_ft)
Updated Name: Lake Pontchartrain
Updated depth: 65
Tip
Basic rules for variable names:
Variables cannot start with a number
Variables can contain letters (a-z, A-Z), numbers (0-9), and underscores (_)
Variables are case-sensitive, so myVariable and myvariable would be considered different variables
Multiple variables#
You can assign multiple values (by separating the values with commas) to multiple variables simultaneously.
# Multiple assignment
satellite, launch_year, weight = "Landsat8", 2013, 2071.5
print("Satellite:", satellite)
print("Launch year:", launch_year)
print("Weight:", weight)
Satellite: Landsat8
Launch year: 2013
Weight: 2071.5
You can unpack multiple values from a list or tuple to multiple variables.
# Unpacking
values = ("Landsat8", 2013, 2071.5)
satellite, launch_year, weight = values
print("Satellite:", satellite)
print("Launch year:", launch_year)
print("Weight:", weight)
Satellite: Landsat8
Launch year: 2013
Weight: 2071.5
Important
The number of variables on the left side of the assignment operator must match the number of values in the iterable.